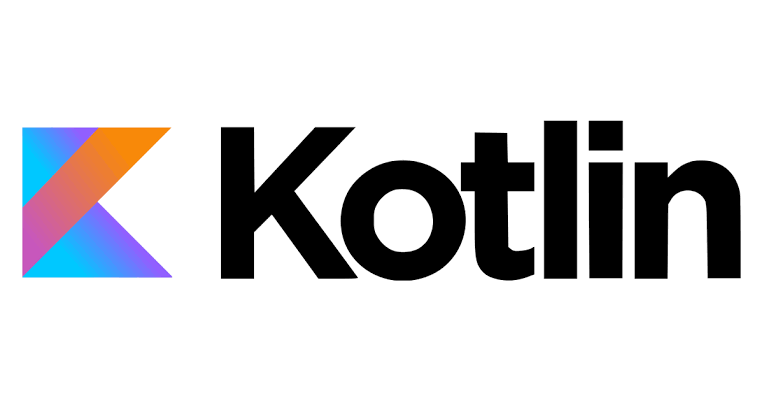
In Kotlin server-side application development, prioritizing robust security measures is paramount. Developers frequently confront a spectrum of threats and vulnerabilities that could potentially compromise the integrity of their applications. This article aims to delve into comprehensive security strategies tailored specifically for Kotlin server applications, furnishing developers with the knowledge to fortify their systems against potential risks.
The scope of security in Kotlin server applications extends beyond thwarting unauthorized access; it encompasses safeguarding against a myriad of threats such as injection attacks, cross-site scripting (XSS), and data breaches.
Within the pages of this article, we will explore fundamental security practices, diverse authentication methods, access control mechanisms, input validation strategies, secure communication protocols, and more.
Authentication Mechanisms:
Implementing robust authentication mechanisms in Kotlin server applications is crucial for controlling access and ensuring data security. One popular method is token-based authentication, utilizing tokens like JSON Web Tokens (JWTs) for user verification. This approach offers scalability and flexibility, enabling stateless authentication.
An illustrative example of JWT-based authentication in Kotlin using the java-jwt library is as follows:
// Code example for generating JWT token
import com.auth0.jwt.JWT
import com.auth0.jwt.algorithms.Algorithm
import java.util.*
fun generateToken(userId: String): String {
val algorithm = Algorithm.HMAC256("secret_key")
return JWT.create()
.withIssuer("your_issuer")
.withSubject(userId)
.withExpiresAt(Date(System.currentTimeMillis() + 3600000))
.sign(algorithm)
}
This function generates a JWT token for a given user ID, signed using an HMAC algorithm. It’s imperative to securely manage the secret key.
Authorization and Access Control:
Authorization involves defining and managing user roles and permissions to regulate access to different parts of an application. In Kotlin, implementing Role-Based Access Control (RBAC) ensures proper access control within the server.
A simple RBAC setup in Kotlin using Ktor could look like this:
// Code example for RBAC setup
enum class UserRole {
ADMIN, USER, GUEST
}
fun checkPermission(userRole: UserRole, resource: String, action: String): Boolean {
return when (userRole) {
UserRole.ADMIN -> true
UserRole.USER -> resource == "profile" && (action == "read" || action == "write")
UserRole.GUEST -> resource == "public" && action == "read"
}
}
In this example, checkPermission()
validates whether a user with a specific role has permission to perform a particular action on a resource.
Input Validation and Data Sanitization:
In Kotlin server applications, meticulous validation and sanitization of user inputs are imperative to prevent security vulnerabilities like SQL injection and XSS attacks. Consider the following example of input validation using regular expressions in Kotlin:
// Code example for input validation
fun isValidEmail(email: String): Boolean {
val emailRegex = "^[A-Za-z](.*)([@]{1})(.{1,})(\\.)(.{1,})"
return email.matches(emailRegex.toRegex())
}
fun sanitizeInput(input: String): String {
return input.replace("<", "<").replace(">", ">")
}
The isValidEmail()
function uses a regular expression to verify if an email address is in the correct format. Meanwhile, sanitizeInput()
escapes HTML tags to prevent XSS attacks.
Secure Communication and HTTPS:
Securing communication between clients and servers is imperative to protect sensitive data. Enabling HTTPS (HTTP Secure) ensures encrypted data transmission over the network. In Kotlin server applications, configuring HTTPS can be achieved using Ktor:
// Code example for configuring HTTPS in Ktor
import io.ktor.server.engine.embeddedServer
import io.ktor.server.netty.Netty
import io.ktor.features.HSTS
import io.ktor.features.HttpsRedirect
fun main() {
val server = embeddedServer(Netty, port = 8080) {
install(HttpsRedirect) {
sslPort = 8443
}
install(HSTS) {
includeSubDomains = true
}
}
server.start(wait = true)
}
In this example, Ktor’s HttpsRedirect
feature redirects HTTP requests to HTTPS, ensuring secure communication. Ensure that your project has the necessary Ktor dependencies and that you’ve imported the required features (HSTS and HttpsRedirect) to use these functionalities.
Handling Security Headers and CSRF Protection:
Security headers play a crucial role in mitigating various web vulnerabilities. Configuring headers like Content Security Policy (CSP) and X-Frame-Options is essential in Kotlin server applications to enhance security. Consider the following example of implementing security headers using Ktor:
// Code example for implementing security headers in Ktor
import io.ktor.application.*
import io.ktor.features.*
fun Application.installSecurityHeaders() {
install(DefaultHeaders)
install(ContentNegotiation)
install(Compression)
install(CachingHeaders)
install(XForwardedHeaderSupport)
install(FrameOptions) {
frameOptionsHeader = "DENY"
}
install(ContentSecurityPolicy) {
default {
frameAncestors {
self
}
script {
unsafeInline = ContentSecurityPolicyHeader.UnsafeInlineSource
}
}
}
}
In this example, Ktor’s ContentSecurityPolicy
and FrameOptions
features are used to define security headers like Content Security Policy and X-Frame-Options, mitigating potential risks like clickjacking attacks.
Logging and Monitoring for Security:
Comprehensive logging is crucial for detecting security incidents and analyzing application behavior. Logging security-related events and suspicious activities help identify potential threats in Kotlin server applications. Consider the following example of implementing logging using the Logback logging framework in Kotlin:
// Code example for implementing logging in Kotlin using Logback
import org.slf4j.LoggerFactory
val logger = LoggerFactory.getLogger("SecurityLogger")
fun logSecurityEvent(event: String) {
logger.info("Security event: $event")
}
In this example, a SecurityLogger
instance is created using Logback to record security events such as authentication failures or access denied attempts.
Securing Dependencies and Patch Management:
Maintaining the security of dependencies within Kotlin projects is fundamental to preempting vulnerabilities introduced by third-party libraries. Regularly updating and managing dependencies is critical to addressing potential security flaws that could compromise the application’s integrity.
To ensure the security of dependencies, it’s vital to employ best practices such as version pinning and utilizing tools like Gradle or Maven. Consider the following practices for securing dependencies in Kotlin projects:
Version Pinning:
Defining specific versions of dependencies in project configuration files (e.g., build.gradle.kts for Gradle or pom.xml for Maven) ensures that known vulnerabilities are patched. By explicitly specifying versions, developers can control which libraries and versions their project utilizes, minimizing exposure to security risks associated with outdated or vulnerable dependencies.
Dependency Update Automation:
Leveraging automated tools or services that periodically scan for dependency updates and security vulnerabilities (e.g., Dependabot, Renovate) can significantly streamline the process of managing dependencies. These tools automatically identify outdated dependencies and suggest updated versions or patches, simplifying the task of keeping dependencies secure and up-to-date.
In Kotlin projects, managing dependencies securely involves explicitly declaring libraries and versions in build configuration files:
Gradle (build.gradle.kts):
// Code example for managing dependencies in Gradle
dependencies {
implementation("org.jetbrains.kotlin:kotlin-stdlib:1.6.0")
}
Maven (pom.xml):
<!-- Code example for managing dependencies in Maven -->
<dependencies>
<dependency>
<groupId>org.jetbrains.kotlin</groupId>
<artifactId>kotlin-stdlib</artifactId>
<version>1.6.0</version>
</dependency>
<!-- Add other dependencies securely and define versions -->
</dependencies>
By employing these practices and utilizing tools that aid in dependency analysis and updates, Kotlin projects can maintain a more robust and secure ecosystem, mitigating the risks associated with outdated or vulnerable dependencies.
Testing and Secure Development Practices:
Security testing plays a pivotal role in identifying vulnerabilities within Kotlin server applications. Adopting various testing methodologies helps in detecting and rectifying security flaws early in the development lifecycle.
Penetration Testing:
Performing penetration tests involves simulating attacks to assess the system’s security posture. Tools like OWASP ZAP or Burp Suite assist in identifying potential vulnerabilities like SQL injection, XSS, or CSRF.
Vulnerability Scanning:
Automated vulnerability scanning tools, such as Nessus or OpenVAS, can help you identify security weaknesses within the application and its dependencies. These tools scan for known vulnerabilities, outdated libraries, or misconfigurations.
Conclusion:
Throughout this comprehensive guide, we’ve explored diverse security measures tailored specifically for Kotlin server applications. From authentication mechanisms to securing communication, input validation, and compliance considerations, each aspect contributes significantly to fortifying the application against potential threats.
Ensuring the security of Kotlin server applications is an ongoing endeavour. It’s crucial to emphasize the need for continuous vigilance, proactive monitoring, and a security-first approach to address evolving security challenges.
To create secure Kotlin applications, adopting a security-first mindset is imperative. Prioritizing security considerations at every stage of the development lifecycle helps in crafting robust and trustworthy server applications.
By implementing the best practices discussed in this guide and staying updated on emerging security trends, you can significantly enhance the security of your server applications.
We provide comprehensive information about our services to help you make the best choice for your needs. Take your time to browse through our website and feel free to reach out if you have any questions.